Note
Go to the end to download the full example code.
Manipulating Skim matrices#
In this example we show how to open and manipulate skim matrices
Imports#
from pathlib import Path
import numpy as np
from polaris import Polaris
Data Sources#
Open the demand database for analysis
model_fldr = "/tmp/Grid"
project = Polaris.from_dir(Path(model_fldr))
highway_skim = project.skims.highway
pt_skim = project.skims.transit
We can see what intervals exist in a matrix
print(highway_skim.intervals)
[240, 360, 420, 480, 540, 600, 720, 840, 900, 960, 1020, 1080, 1140, 1200, 1320, 1440]
For accessing costs, distances and time can be done in two different ways for highway skims
time240 = highway_skim.time[240]
distance240 = highway_skim.distance[240]
cost240 = highway_skim.cost[240]
dist_skim = highway_skim.get_skims(interval=480, metric="distance")
For PT skims there is only one way
time_pt_240 = pt_skim.get_skims(interval=240, mode="Bus", metric="time")
We can also plot a histogram (Distance frequency distribution)
import matplotlib.pyplot as plt
c, x = np.histogram(np.ravel(dist_skim), bins=50, density=True)
plt.plot(x[:-1], c)
plt.title("Distribution of auto distance skim for 8am")
plt.xlabel("Distance (m)")
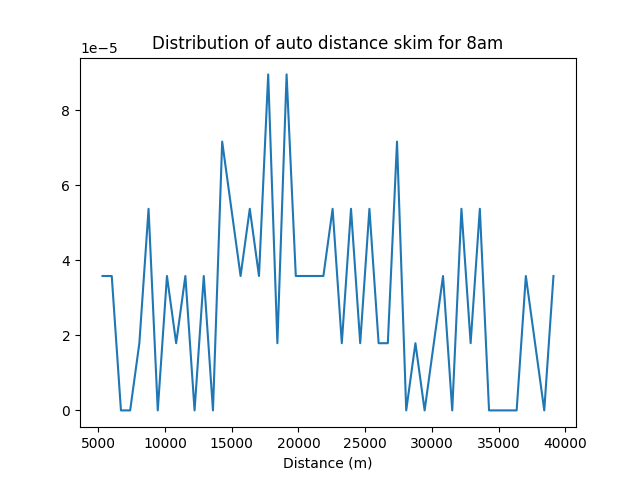
Text(0.5, 23.52222222222222, 'Distance (m)')
For PT skims we can list modes and metrics as well
print(pt_skim.intervals)
print(pt_skim.modes)
print(pt_skim.metrics)
[240, 360, 420, 480, 540, 600, 720, 840, 900, 960, 1020, 1080, 1140, 1200, 1320, 1440]
['BUS', 'RAIL', 'PARK_AND_RIDE', 'PARK_AND_RAIL', 'RIDE_AND_UNPARK', 'TNC_AND_RIDE']
['time', 'walk_access_time', 'auto_access_time', 'wait_time', 'transfers', 'fare']
We can remove intervals for the skim matrix
highway_skim.remove_interval(1020)
pt_skim.remove_interval(1020)
We can also add new skims to the file
First with ZEROS
# For highway skims
highway_skim.add_interval(1000)
print("Highway intervals", highway_skim.intervals)
print("Highway matrix sum", highway_skim.time[1000].sum())
# For PT skims
pt_skim.add_interval(1010)
print("Transit intervals", pt_skim.intervals)
print("Transit matrix sum", pt_skim.get_skims(interval=1010, mode="Bus", metric="time").sum())
Highway intervals [240, 360, 420, 480, 540, 600, 720, 840, 900, 960, 1000, 1080, 1140, 1200, 1320, 1440]
Highway matrix sum 0.0
Transit intervals [240, 360, 420, 480, 540, 600, 720, 840, 900, 960, 1010, 1080, 1140, 1200, 1320, 1440]
Transit matrix sum 0.0
Or by copying another interval
# For Highways
highway_skim.add_interval(1350, copy_interval=1320)
print("Highway intervals", highway_skim.intervals)
print("The time skim totals match:", highway_skim.time[1320].sum(), highway_skim.time[1350].sum())
print("The distance skim totals also match:", highway_skim.distance[1320].sum(), highway_skim.distance[1350].sum())
print("The cost skim totals also match:", highway_skim.cost[1320].sum(), highway_skim.cost[1350].sum())
# For PT
pt_skim.add_interval(1390, copy_interval=1320)
print("Transit intervals", pt_skim.intervals)
print(
"The time skim totals match:",
np.nansum(pt_skim.get_skims(interval=1390, mode="Bus", metric="time")),
np.nansum(pt_skim.get_skims(interval=1320, mode="Bus", metric="time")),
)
Highway intervals [240, 360, 420, 480, 540, 600, 720, 840, 900, 960, 1000, 1080, 1140, 1200, 1320, 1350, 1440]
The time skim totals match: 1574.3708 1574.3708
The distance skim totals also match: 1596717.8 1596717.8
The cost skim totals also match: 0.0 0.0
Transit intervals [240, 360, 420, 480, 540, 600, 720, 840, 900, 960, 1010, 1080, 1140, 1200, 1320, 1390, 1440]
The time skim totals match: inf inf
Or by copying another interval Since all the changes we made to the matrices only live in memory, we write them down to disk
hwy_skim_file = Path("/tmp/new_highway_skim.omx")
pt_skim_file = Path("/tmp/new_pt_skim.omx")
hwy_skim_file.unlink(missing_ok=True)
pt_skim_file.unlink(missing_ok=True)
pt_skim.write_to_file(pt_skim_file)
highway_skim.write_to_file(hwy_skim_file)
Total running time of the script: (0 minutes 1.198 seconds)