Paths#
Paths (also called trajectories) are written to the H5 file for a sub-set of the total trips which were made during
simuation according to parameter vehicle_trajectory_sample_rate
. Generally this is set to approximately 1% of the
total population.
In order to work with Path data, it is recommended to use the H5_Results
class to extract the raw data and make it
easier to manipulate.
from polaris.runs.results.h5_results import H5_Results
results = H5_Results("~/models/Bloomington/Bloomington-Result.h5")
paths = results.load_paths()
This will return a single pandas DataFrame with all the paths that are available across the entire simulation. This
includes only high level metadata on the paths, not the actual link sequences traversed. To get the link sequences
use the load_path_links
method:
path_links = results.load_path_links() # Load link sequences for all paths
path_link_17 = results.load_path_links(path_id=17) # Load link sequences for just path 17
The path_id
corresponds to the path_id
column in the Trip table in the Demand database.
Multi-modal paths#
Multi-modal paths attributes and the associated detailed link trajectories are also stored in the H5 file and can be retreieved similarly to the regular paths.
paths = results.get_mm_paths()
paths[paths.link_first_index > 0]
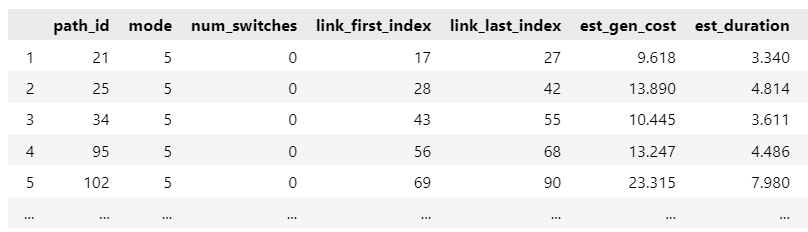
result.get_path_mm_links(21)
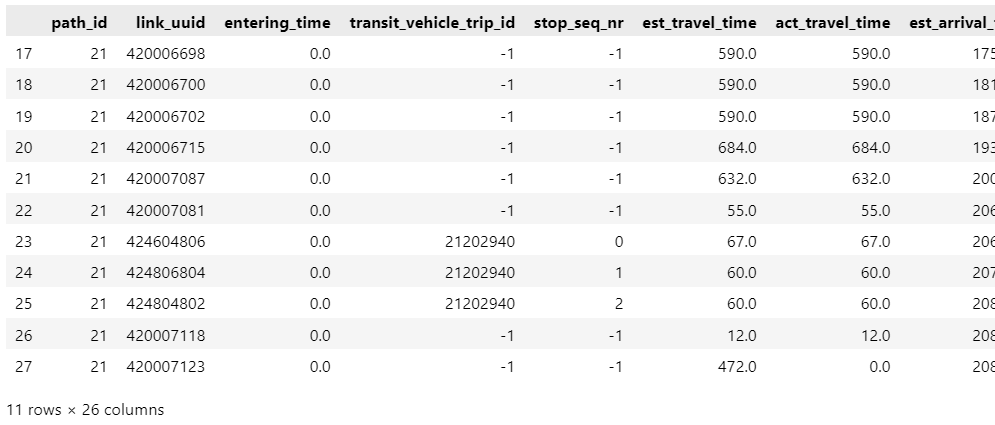
Table structure#
Futher detail on the actual structure of each table stored in the paths
group is provided here.
All tables are suffixed with the time-step at which they were written (in seconds from midnight). For example the path_timestep_14400
contains the high level records for each path what was made between midnight and 4am (4 * 60 * 60 = 14400 seconds) - it will be paired with a corresponding path_links_timestep_14400
and a path_units_timestep_14400
table which contain the more detailed link and unit records respectively.
As all records have to be stored internally as integers, we use a strategy of multiplying values by 1,000 when inserting them into the H5 and dividing them by 1,000 when extracting them. Thus the actual unit stored internally is shifted (meters becomes millimeters, for example). In the below tables the outer unit is given.
Path#
Column Name |
Description |
---|---|
path_id |
Unique identifier for this path |
link_first_index |
The index (within the set of links for the current timestep) of the first link in the sequence |
link_last_index |
The index (within the set of links for the current timestep) of the last link in the sequence |
link_first_index |
The index (within the set of units for the current timestep) of the first unit in the sequence |
link_last_index |
The index (within the set of units for the current timestep) of the last unit in the sequence |
Path Links#
This table stores the travel time and energy consumption of a vehicle on a link by link basis.
Column Name |
Description |
---|---|
path_id |
Identifier for the path that this record belongs to |
link_uid |
UUID of the current link in the path sequence |
entering_time |
Time at which this link was entered (units: seconds) |
travel_time |
Actual travel time to traverse this link (units: seconds) |
energy_consumption |
The energy consumed by the vehicle while traversing this link (units: Wh) |
routed_travel_time |
Estimated travel time to traverse this link (units: seconds) |
Path Units#
This table stores the position and velocity data of a vehicle on a second by second basis.
Column Name |
Description |
---|---|
path_id |
Identifier for the path that this record belongs to |
sim_index |
The timestep at which this record was taken |
link_uid |
UUID of the current link in the path sequence |
speed |
Speed at which the vehicle was moving (units: m/sec) |
position |
Position along the current link (units: meter) |
Path MultiModal#
This table contains records for each multimodal trajectory that was undertaken in the simulation. Unlike the path table above, multimodal path attributes are written for all records without sub-sampling, however sub-sampling is used to reduce the actual number of link-specific detailed trajectories stored in the path multimodal links table (see below). Note that both router-estimates and experienced values are logged in this tables with the prefix est_
and act_
respectively.
Column Name |
Description |
---|---|
path_id |
Unique identifier for this multimodal path |
mode |
Mode identifier for trips (e.g., !Vehicle_Type_Keys!) |
Number_Of_Switches |
Total number of reroutes/detours that occurred during path execution |
link_first_index |
The index (within the set of links for the current timestep) of the first link in the sequence |
link_last_index |
The index (within the set of links for the current timestep) of the last link in the sequence |
Gen_Cost |
Generalized cost for this path |
Duration |
Time taken to traverse this multimodal link (units: seconds) |
Arrival_Time |
The time at which this path finished traversing the last link (units: seconds) |
Bus_Wait_Time |
Wait time to board a bus. Can be 0 if bus is not the next transfer. (units: seconds) |
Rail_Wait_Time |
Wait time to board a rail. Can be 0 if rail is not the next transfer. (units: seconds) |
Comm_Rail_Wait_Time |
Wait time to board a commuter rail. Can be 0 if commuter rail is not the next transfer. (units: seconds) |
Walk_Time |
Walk time along this link (units: seconds) |
Bike_Time |
Bike time along this link (units: seconds) |
Bus_IVTT |
Bus in-vehicle travel time along this link (units: seconds) |
Rail_IVTT |
Rail in-vehicle travel time along this link (units: seconds) |
Comm_Rail_IVTT |
Commuter rail in-vehicle travel time along this link (units: seconds) |
Car_Time |
Auto in-vehicle travel time along this link (units: seconds) |
Wait_Count |
Number of transfers |
Transfer_Pen |
Total transfer penalty incurred in traversing the path (units: seconds) |
Standing_Pen |
Total penalty for standing in a crowded transit mode incurred in traversing the path (units: seconds) |
Capacity_Pen |
Estimated capacity penalty (using CapacityAlpha from MultimodalRouting.json) when load exceeds a threshold (units: seconds) |
Monetary_Cost |
Monetary cost that is incurred in traversing the path (units: $USD) |
TNC_Wait_Count |
Number of transfers to and from a TNC mode |
TNC_Wait_Time |
Wait time to board a TNC Vehicle. Can be 0 if TNC is not the next transfer. (units: seconds) |
Path MultiModal Links#
This table contains records for each link along a multimodal trajectory. Generally only a sub-set of the multimodal paths found in the path_multimodal table are actually written out in detail to this table. Link-specific router-estimates and experienced values are logged in this tables with the prefix est_
and act_
respectively.
Column Name |
Description |
---|---|
path_id |
Unique identifier for this multimodal path |
link_uid |
UUID of the current link in the path sequence |
entering_time |
The time at which the traveler entered this link. (units: seconds) |
transit_vehicle_trip_id |
The transit vehicle trip id of the boarded vehicle (-1 if not applicable) |
stop_seq_nr |
The stop sequence number at which the transit vehicle was boarded (-1 if not applicable) |
travel_time |
Travel time along this link (units: seconds) |
arrival_time |
The time at which this path finished traversing the last link (units: seconds) |
gen_cost |
Generalized cost incurred by traversing this link |
wait_count |
Boolean indicating if a boarding occurred at the start of this link |
tnc_wait_count |
Boolean indicating if a TNC boarding occurred at the start of this link |
wait_time |
The time which was spent waiting at the start of a link for boarding (units: seconds) |
transfer_penalty |
Total transfer penalty incurred in traversing this link in the path (units: seconds) |
standing_penalty |
Total penalty for standing in a crowded transit mode incurred in traversing this link in the path (units: seconds) |
capacity_penalty |
Estimated capacity penalty (using CapacityAlpha from MultimodalRouting.json) when load exceeds a threshold (units: seconds) |
monetary_cost |
Monetary cost that is incurred in traversing this link in the path (units: $USD) |